CSS selectors
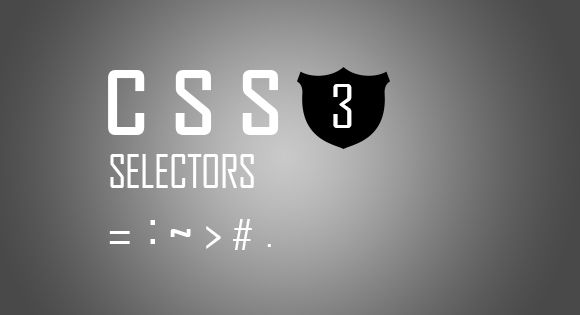
CSS selectors are not a new topic, nor the one to be posted now, but it’s something I’m really interested in and have been working on this for a while.
CSS selectors will not be new to most of us, the more basic selectors are type (div
), ID (e.g. #container
) & class (.sidebox
). Besides the usual type, class and ID selectors, CSS offers several pseudo-class and pseudo-element selectors that allow us to target HTML elements based on their positions in the document, some even target virtual elements and generate content from the CSS. Uncommon ones include the pseudo-classes ( :hover
) and more complex ones such as :first-child
or [class^="grid-"]
.
The key lies in using CSS selectors efficiently to maximize performance and minimize load time, though this efficient usage matters only for website with thousands of DOM elements and the speed is the key for the existence in the market.
CSS selectors
CSS selectors have an inherent efficiency, the order of high to less efficient CSS selectors with examples :
- ID,
#header
- Class,
.promo
- Type,
div
- Adjacent sibling,
h2 + p
- Child,
li > ul
- Descendant,
ul a
- Universal,
*
- Attribute,
[type="text"]
- Pseudo-classes/-elements,
We will get a detailed look at these selectors with an example soon though you many not need it but definitely a newbie will, many of the selectors mentioned in this article are part of the CSS3 specifications, and are only available in modern browsers.
Combining selectors
Image credit: Dennis AB on Flickr
Before we start with selectors i would like to mention a few words on Combining them, and how the browsers interpret it. You can have standalone selectors such as #menu
, which will select any element with an ID of ‘menu’, or you can have combined selectors such as #menu a
which will match any anchors within any element with an ID of ‘menu ‘.
We Humans read these CSS left-to-right. We look out for #menu
and then any a elements inside there. Browsers read these differently; browsers read selectors right-to-left.
When combining more than one selector the right-most part of a larger CSS selector is called key selector. It’s more efficient for a browser to start with the key selector and work its way back up the DOM tree than to start up the DOM tree and take a journey down as it might not even end up at the key selector most of the time.
Where we see a #menu
with an a in it, browsers see an a
in a #menu
. This subtle difference has a huge impact on selector performance, and is a very important to know these.
CSS selectors
ID Selector
Prefixing the hash symbol to a selector allows us to target by id. This is easily the most common usage, however be cautious when using id selectors.
#main{
width: 960px;
margin: 10px auto;
}
ID selectors are rigid and don’t allow reuse. If possible, first try to use a tag name of HTML5 elements, or even a pseudo-class.
Class Selector
Prefixing the period or Full-stop (.) symbol to a selector allows us to target by class. Mainly used to for styling a group of elements.
.red-border{
border:2px solid red;
}
The difference between #id
and a .class
is that, with the latter, you can target multiple elements.
Type Selector
A type selector used to target all elements on a page, according to the type without an id or class.
a { color: red; } // Apply red color to all Anchor tags
ul { margin-left: 0; } // Apply margin-left 0 to all un-ordered list
Adjacent Sibling Selector
An adjacent selector will select only the element that is immediately preceded by the former element. In this case, only the first paragraph after each h2 will have bold text.
h2 + p {
font-weight: bold;
}
Child
Child Selector
A Child selector target the elements which are direct children of the parent element. It will not target Child of the Child elements.
li > ul { color: #fff; }
It will not target, that is all the Child’s of the Child with specified elements will not have the property. Consider the following markup for detail.
A property applied for #menu> ul
will target the direct children ul
of the div
with id menu
. It will not target the ul
that is a child of the first li
.
Descendant Selector
The commonly used selector after .class
is the descendant . Mainly used to increase the specificity.
p a {
text-decoration: none;
}
The above example targets all the anchors inside paragraph tag and removes the decorations. This is allows to select elements more specifically.
Universal Selector
The Universal Selector as the name indicates will target every single element on the page. Developers use this to zero out the margins and padding.
* {
margin: 0;
padding: 0;
}
It is recommended not to use this in production code as it adds extra weight on the browser and is unnecessary. Instead use type selector.
html, body, header, footer, article, nav, section, asi
de, time, h1, h2, h3, p, a, ul, li, dl, dd, dt, table, thead, tfoot, tbody, th, tr, td{
border:0;
margin:0;
outline:0;
padding:0;
}
The * can also be used with child selectors.
Attributes Selector
Attributes selects the tags that have the specified attribute. There are seven type available at present. They are:
- [title]
- [href=”foo”]
- [href*=”nettuts”]
- [href^=”http”]
- [href$=”.jpg”]
- [data-*=”foo”]
- [foo~=”bar”]
[title]
This will only select the anchor tags that has the specified attribute.
a[head] {
color: #fff;
}
[href=”http://nnn”]
The snippet above will style all anchor tags which link to http://nnn .All other anchor tags will remain unaffected.
a[href="http://example.com"] {
color: #000;
}
[href*=”nettuts”]
The star designates that the proceeding value appear somewhere in the attribute’s value.All other anchor tags will remain unaffected as above.
a[href*="example"] {
color: #000;
}
If the anchor tag linked to other domain with the string example in the url, the property will be applied. To be more specific, use ^
and &
, to reference the beginning and end of a string, respectively.
[href^=”http”]
This works similar to the above one but will check whether http is present in the starting of the string, and apply the action. Designers use these to provide give a different style to external links, even add icon.
a[href^="http"] {
color: red;
}
Rest of the Attributes selectors works similar to the above one but with ^
and &
they are more specific.
Pseudo-classes Selector
This pseudo class will only target a user interface element that has been checked – like a radio button, or checkbox and more. It’s as simple as that.The below example gives a green color to anchors when hovered on.
a:hover {
color:green;
}
There is a lot of Pseudo-classes Selector selectors available, i am not mentioning them with an example and description to keep the article length.
- :active
- :hover
- :visited
- :checked
- :after
- :before
- :not(selector)
- ::pseudoElement – To style fragments of an element like first like in a paragraph.
- :nth-child(n) – To target nth child element.
- :nth-last-child(n) – Again to target nth child element but from the end.
- :first-child
- :last-child
Effective Utilization Of CSS Selectors
With the Knowledge of how browser interpret the CSS , we can look a step further to optimize them for speed. The best thing about having nice explicit key selectors is that you can often avoid over qualifying selectors. Which looks like:
html body .main #menu a{}
There is just too much in there, and at least 3 of these selectors are totally unnecessary. The same purpose can be achieved with this
#menu a{}
Well in the first one, that the browser has to look for all a elements, then check whether they’re in the element with an ID of ‘menu’, so on right the way up to the html. This creates too many condition checks that we really don’t need.
Overqualified selectors make the browser work harder than it needs to and uses up its time; increase the browser performance by cutting the unnecessary bits out.
Conclusion
With a load of CSS selectors its easier to design a website at easy than earlier, but If you’re compensating for older browsers, like IE 6, you still need to be careful when using these newer selectors. But, please don’t let that deter you from learning these. You can use external script to bring support for these selectors to older browsers. Always recommend your users to upgrade their browser.
Always try to use these native CSS3 selectors when using Jquery, It’ll make your code faster, and let it use the browser’s native parsing.
Thank you for taking you time to read one of my longest article. You are welcome to express views via comments.