CSS Drop down menu
In this tutorial we’ll create a Drop down menu with CSS and Java Script.
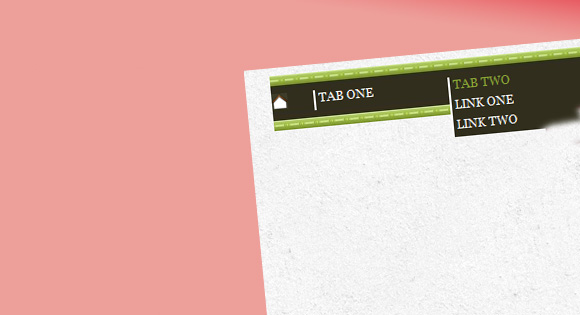
The Following example will only work with the following browsers only.
Here is a css drop down menu that drops down on mouse over, it uses a simple image for background (you can remove it and use a colour instead or leave it blank) , and a simple java script.
The Mark Up
The CSS
/* menu */
p#menuHome {
float:left;
margin-top:0px;
margin-right: 20px;
}
p#menuHome a {
display: block;
width: 16px;
height: 16px;
background-image: url(../images/home_white_16.png);
background-repeat: no-repeat;
}
p#menuHome a:hover {
background-image: url(../images/home_green_16.png);
}
#menuTop {
position: relative;
background-image: url(../images/navbkgd.jpg);
// the back groud image
background-repeat: repeat-x;
padding-top: 18px;
height: 40px;
margin-top:-15px;
font-size: 9pt;
width: auto;
}
#menuTop ul, #menuTop li {
margin: 0;
padding: 0;
}
#menuTop ul {
list-style-type: none;
}
#menuTop li {
display: block;
background-color: #312e1d;
width: 130px;
height: 20px;
margin: 0 3px 0 0;
padding: 0;
border-left: 2px solid white;
}
#menuTop a {
display: block;
margin: 0;
padding: 0 3px;
}
#menuTop a:link, #menuTop a:visited {
font-family: Georgia, Times, serif;
color: white;
text-decoration: none;
}
#menuTop a:hover {
color: #8aa635;
}
ul.menuHoriz li {
float: left;
}
ul.menuVert {
position: absolute;
visibility: hidden;
top: 38px;
}
/* ul#menuSubOne { left: 0 } */
ul#menuSubOne { left: 234px }
ul#menuSubTwo { left: 369px }
ul#menuSubThree { left: 504px }
ul#menuSubFour { left: 639px }
The Java script
// configuration variables
var timeout = 250; // milliseconds
var fadeSpeed = 500; // milliseconds
var useFade = true;
// timers array
var timers = new Array();
// state array -- by id, value = active, false = inactive
var state = new Array();
// lastOpacity: used to prevent multiple timers from making the
fade flicker
var lastOpacity = new Array();
// MSIE has its own way of setting opacity, so we have to
detect it
// all the other major browsers support the standard DOM
opacity property
var msie = false;
if( navigator.appName == "Microsoft Internet Explorer" ) msie = true;
// entry point: set element to visible and clear its timers
function setMenu( id )
{
var e = document.getElementById(id);
e.style.visibility = "visible";
state[id] = true;
setOpacity( id, 1 );
if(timers[id]) {
clearTimeout(timers[id]);
timers[id] = undefined;
}
}
// set element to hidden and reset its opacity
// typically called by a timer
// may be used as an entry point to bypass timers and fades
function hideMenu( id )
{
var e = document.getElementById(id);
state[id] = false;
e.style.visibility = "hidden";
if(useFade) setOpacity( id, 1 );
}
// entry point: hide the menu using fade (if enabled)
function clearMenu( id )
{
if(useFade) timers[id] = setTimeout( 'fadeMenu( "' + id + '" )', timeout );
else timers[id] = setTimeout( 'hideMenu( "' + id + '" )', timeout );
}
// set the opacity
// special support for MSIE
function setOpacity( id, value )
{
var e = document.getElementById(id);
if(state[id]) value = 1; // menu fade was interrupted
else if(lastOpacity[id] && (lastOpacity[id] < value))
value=lastOpacity[id];
// prevents flicker if multiple timers set
if(msie) e.style.filter = 'alpha(opacity=' + value*100 + ')';
// MS Internet Explorer
else e.style.opacity = (value);// Everyone else (standard DOM)
if( value == 0 ) hideMenu( id );
// when all faded, reset the menu state
lastOpacity[id] = value;
}
// fade a menu
// typically called by a timer
function fadeMenu ( id )
{
var start = 0;
var end = 0;
var s = Math.round( fadeSpeed / 25 ); // fade in 25ms increments
var timer = 0;
var i;
state[id] = false;
for( i = s; i >= 0 ; i-- ) {
setTimeout( "setOpacity('" + id + "'," + ( i / s ) + ")",
timer++ * fadeSpeed / s )
}
}